Action editor for vsAction
The Action Editor panel is used to define actions and how the Action Actors – these are nodes in the IIZI transaction framework (TXP) – are to be ordered and which ones to use or connect to. This definition makes up the TXP process. See section Java class and method connections.
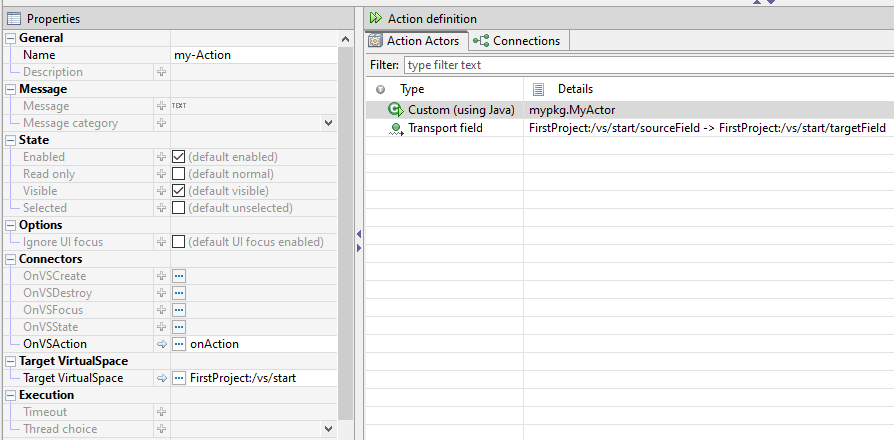
VirtualSpace and its four component types
For simple actions requiring just Java code, you can use the OnVSAction
method when you have specified a Java class that is attached to the namespace, e.g. the namespace is called start
and the vsAction my-Action
is connected to the method onAction
as:
package mypkg;
import com.iizix.api.vs.OnVSAction;
import com.iizix.api.vs.VSActionEvent;
import com.iizix.api.vs.VirtualSpace;
@VirtualSpace(ref = "FirstProject:/vs/start")
public class StartNameSpaceListener {
@OnVSAction(name = "my-Action")
public void onAction(VSActionEvent event) {
// Code here...
}
}
Use the more …
button to drop-down a menu to help you create the method. When you rename the VS Action
, it will update you Java source code (name= “newName”
), and if you refactor the method name using Java Refactoring, the OnVSAction definition will be set to the new name.
The Execution can also be specified to set a Timeout for the TXP process, and in the Thread choice defines in what thread it should execute in. The UI may be locked immediately when the action begins with Lock UI and when it ends using Unlock UI
. If the UI is locked, it must be unlocked somehow; otherwise the client will keep on displaying an “hourglass”. This does not have to be when the action ends: it is up to you when and how to do it (the AppSessionGyro contains methods for the UI lock state).
Action Actors
The Action Actors are defined for a VS Action to make up the TXP process. Each actor is a TXP node, and the ordering is important. The process either completes successfully or rolls back. A failure to complete the process is logged in the server application log as well as to present the error in the UI.
The action actors that can be added are the ones you have created in the various data connectors, a special Java actor and the predefined actors:
Predefined actor | Description |
---|
SetFocus | Set focus |
SetSourceFieldValue | Set source field value |
SetTargetFieldValue | Set target field value |
TransportField | Transports a field |
TransportGroup | Transports a group of components |
TransportSelectedTableRows | Transport selected table rows |
TransportSingleSelectedTableRow | Transport single-selected table row |
TransportSingleSelectedTableRow-AndColumnToField | Transport single-selected table row and colum to field |
TransportSingleSelectedTableRowToFields | Transport single-selected table row to fields |
TransportTableColumn | Transport table column |
TransportTableRows | Transport table rows |
| |
Each actor can provide (and generally do provide) editable properties that needs to be filled in. | |
The VS Action has a property Target namespace that is used for the transport
operations and the SetTargetFieldValue
operation.
Java Action Actor
This actor is used when the Java code needs to execute in the TXP process as a node, i.e. it can handle commit and rollback, etc. The actor is a class that must extend
com.iizix.actionactor.AbstractJavaActionActor
/**
* The Java Action Actor that takes a String parameter
* and a LocalTime Value for show.
*/
@JavaActionActor(descr = "A simple Java Actor without rollback",
modifiesVS = false,
ref = "FirstProject:/vs/start/my-Action")
@JavaActorParam(name = "myString", prompt = "myString for show",
type = JavaActorParam.Type.String)
@JavaActorParam(name = "myTime", prompt = "myTime for show",
type = JavaActorParam.Type.Value_LocalTime,
defaultInput = "00:00")
public class MyActor extends AbstractJavaActionActor {
/**
* Called when the TXP executes this Action Actor node.
*/
@Override
public void onAction(VSActionTXProcess process) {
// Place your code here...
}
}
The class above is an action actor that is connected to the my-Action
VS Action, and prompts the developer to fill in two parameters myParam
as a String and myTime
as a ValueProp of the type LocalTime with a default value of 00:00
. The dialog box presented to the developer looks like:
The feature of making it possible to store parameters for an individual Java Action Actor
is very useful as it allows a class to be re-used in various places where the parameters can be used to set the context for the class instance. See the JavaDoc
of the @JavaActorParam
for additional annotation parameters such as allowNull, tooltip, etc.
The Refresh button in the dialog may be used if the parameters do not match the class if you change the class name. Pressing Refresh
will reload the class annotations and refresh the properties displayed.